Iterative Prediction of Spring-Mass System
claimed by kgiles7
The Main Idea
A simple spring-mass system is a basic illustration of the momentum principle. The principle of conservation of momentum can be repeatedly applied to predict the system's future motion.
A Mathematical Model
The Momentum Principle provides a mathematical basis for the repeated calculations needed to predicts the system's future motion. The most useful form of this equation for predicting future motion is referred to as the momentum update form, and can be derived by rearranging the Momentum Principle as shown below:
[math]\displaystyle{ {{Δp}_{system}} = {\vec{F}_{net}{Δt}} }[/math]
[math]\displaystyle{ {\vec{p}_{f} - \vec{p}_{i} = \vec{F}_{net}{Δt}} }[/math]
[math]\displaystyle{ {\vec{p}_{f} = \vec{p}_{i} + \vec{F}_{net}{Δt}} }[/math]
Velocity Update Formula: [math]\displaystyle{ {\vec{v}_{f} = \vec{v}_{i} + \frac{\vec{F}_{net}}{m}}{Δt} }[/math]
Position Update Formula: [math]\displaystyle{ {\vec{r}_{f} = \vec{r}_{i} + \vec{v}_{avg}{Δt}} }[/math]
A Computational Model
By definition, iterative prediction requires repeated calculations. Computations that require significant time and attention to detail when performed by hand can be done incredibly quickly and accurately by a computer model.
Coding iterative prediction is very similar to writing equations by hand. The same approach is used; the largest difference arises in syntax, as coding requires variables and calculations to be written in a specific format that varies depending on the program used. In the following discussion, VPython will be used to illustrate a computational model of iterative prediction of a spring-mass system.
The text in italics is valid Python code. Notes in regular text to the right the hashtag (#) on each line are explanatory notes that are not part of the code. The complete program, which includes some visual aspects not described below, can be viewed here.
1) Determine initial parameters and constants
The required variables will vary depending on the problem but a general example is provided below:
g = 9.8 ##gravitational constant
mball = .5 ##mass of ball
L0 = 0.3 ##relaxed length of spring
ks = 50 ## spring constant (in N/m)
deltat = .001 ## time step
t = 0 ## initial time = 0 seconds
velocity = (-0.1, 0.2, 0.4)
2) Create objects
Create visual representations of the spring and mass attached to it
ceiling = box(pos=vec(0,0,0), size=vec(0.2,0.01,0.2))
The initial position of the ball is indicated here. An arbitrary size and color have been assigned.
ball = sphere(pos=vec(-.1,-.1,-.1), radius=0.025, color=color.orange)
The spring is attached to the ceiling, as indicated by "pos=ceiling.pos". Again, an arbitrary color and size, as well as number of coils have been assigned to it.
spring = helix(pos=ceiling.pos, color=color.cyan, thickness=.003, coils=40, radius=0.015)
The spring should extend from the point of attachment (ceiling,pos) to the position of the ball (ball.pos), so the next line of code should be included as well.
spring.axis = ball.pos - ceiling.pos
3) Calculate initial momentum
Find the initial momentum by calculating the product of the velocity vector and mass of the ball.
ball.p = mball*velocity
4) Begin calculation loop
This will include all equations that must be repeated. Begin by specifying a terminating condition for the loop. This usually consists of an end time, but does not have to.
while t < 10
5) Calculate initial forces acting on system, and sum to find net force
Calculate the spring force using Hooke's Law:
l = ball.pos - ceiling.pos ## calculate the length of the spring
s = l.mag - L0 ## stretch (s) is equal to the difference in the length of the spring (l) and the relaxed length (L0)
lhat = l/l.mag ## determine L(hat)
fspring = ks * s * -lhat ##apply Hooke's Law
Calculate the gravitational force
fgrav = mball*vector(0, -g, 0)
Calculate the net force
fnet = fspring + fgrav
6) Update object's momentum
Apply the momentum principle
ball.p = ball.p + fnet*deltat
5) Update object's velocity
ball.v = ball.p/mball
6) Update object's position
ball.pos = ball.pos + ball.v*deltat
7) Update time
t = t + delta t
8) Repeat calculations
VPython will continue iterating through the calculations included in the loop until the terminating condition is met.
For complete visual code, click here.
Examples
Simple Example
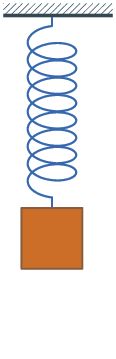
The simplest example of a spring mass system is one that moves in only one-direction.
Consider a massless spring of length 1.0 m with spring constant 40 N/m. If a 10 kg mass is released from rest while the spring is stretched downward to a length of 1.5 m, what is it's position after 0.2 seconds? The mass oscillates vertically, as shown to the right.
Step 1: Set Parameters Before we begin, we must set some parameters that allow the problem to be solved. Since all movement is in the vertical direction, no vector calculation is needed. However, careful attention must be paid to the direction associated with the object's movement and the forces acting on it. For ease in calculations, assume the origin is the point of attachment of the spring to the ceiling above. AS is customary, the positive y-direction will be up, and the negative-y, down. Additionally, the time step used for each iteration must be small enough that we can assume a constant velocity over the interval, but not so large that solving the problem becomes incredibly time-consuming. An appropriately small time step here is approximately 0.1 seconds.
Step 2: Calculate Initial Values Begin by calculating the object's initial momentum and the sum of the forces acting on it. The initial momentum is simply the product of the initial velocity, which is 0 m/s, as the object is released from rest. The forces acting on the mass are the gravitational force exerted by the Earth and the tension exerted by the spring. The net force can be calculated by summing these two forces.
[math]\displaystyle{ {\vec{p}_{i}} = {{m}\cdot\vec{v}_{i}} }[/math]
[math]\displaystyle{ {\vec{p}_{i}} = {{10kg}\cdot{0m/s}} = {0 Ns} }[/math]
[math]\displaystyle{ {\vec{F}_{grav}} = {mg} }[/math]
[math]\displaystyle{ {\vec{F}_{grav}} = {10kg}\cdot{-9.8 m/s/s} = {-9.8 N} }[/math]
[math]\displaystyle{ {\vec{F}_{spring}} = {-kx} }[/math]
[math]\displaystyle{ {\vec{F}_{spring}} = {-40N/m}\cdot{1.0m-1.5m} = {20 N} }[/math]
[math]\displaystyle{ {\vec{F}_{net}} = {\vec{F}_{grav} +{\vec{F}_{spring}}} }[/math]
[math]\displaystyle{ {\vec{F}_{net}} = {-9.8N} +{20 N} = {10.2} }[/math]
Step 3: Update Momentum (Iteration 1)
Using the momentum update formula, calculate the momentum of the mass at the end of the given time step.
[math]\displaystyle{ {\vec{p}_{f} = \vec{p}_{i} + \vec{F}_{net}{Δt}} }[/math]
[math]\displaystyle{ {\vec{p}_{f} = {0Ns} + {10.2N}\cdot{0.1s} = {1.02Ns}} }[/math]
Step 4: Update Velocity (Iteration 1)
[math]\displaystyle{ {\vec{v}_{f} = \vec{v}_{i} + \frac{\vec{F}_{net}}{m}}{Δt} }[/math]
[math]\displaystyle{ {\vec{v}_{f} = {0m/s} + \frac{1.02N}{1 kg}}{0.1s} = {0.102m/s} }[/math]
Step 5: Update Position (Iteration 1)
Using the position update formula, calculate the position of the mass at the end of the given time step. The average velocity used below is the velocity of the mass at the end of the time step. As with all simplifying assumptions, this measurement is not exact. However, it allows for a close enough approximation that the results derived are still valid.
[math]\displaystyle{ {\vec{r}_{f}} = {\vec{r}_{i} + \vec{v}_{avg}{Δt}} }[/math]
[math]\displaystyle{ {\vec{r}_{f}} = {-1.5m} + {0.102m/s}{0.1s} = {-1.398m} }[/math]
Step 6: Update Time (Iteration 1)
[math]\displaystyle{ {\vec{t}_{f}} = {\vec{t}_{i}} + {Δt} }[/math]
[math]\displaystyle{ {\vec{t}_{f}} = {0s} + {0.1s} = {0.1s} }[/math]
Step 7: Repeat Calculations
Repeat the above calculations using the "Iteration Round 1 Final Values" (calculated above) as the "Iteration Round 2 Initial Values".
Step 8: Update Forces (Iteration 2)
The gravitational force on the mass remains constant, and does not need to be recalculated.
[math]\displaystyle{ {\vec{F}_{spring}} = {-kx} }[/math]
[math]\displaystyle{ {\vec{F}_{spring}} = {-40N/m}\cdot{1.0m-1.398m} = {15.92 N} }[/math]
[math]\displaystyle{ {\vec{F}_{net}} = {\vec{F}_{grav} +{\vec{F}_{spring}}} }[/math]
[math]\displaystyle{ {\vec{F}_{net}} = {-9.8N} +{15.92 N} = {6.12N} }[/math]
Step 9: Update Momentum (Iteration 2)
[math]\displaystyle{ {\vec{p}_{f} = \vec{p}_{i} + \vec{F}_{net}{Δt}} }[/math]
[math]\displaystyle{ {\vec{p}_{f} = {1.02Ns} + {6.12N}\cdot{0.1s} = {1.632Ns}} }[/math]
Step 10: Update Velocity (Iteration 2)
[math]\displaystyle{ {\vec{v}_{f} = \vec{v}_{i} + \frac{\vec{F}_{net}}{m}}{Δt} }[/math]
[math]\displaystyle{ {\vec{v}_{f} = {0m/s} + \frac{1.632N}{1 kg}}{0.1s} = {0.1632m/s} }[/math]
Step 11: Update Position (Iteration 2)
[math]\displaystyle{ {\vec{r}_{f}} = {\vec{r}_{i} + \vec{v}_{avg}{Δt}} }[/math]
[math]\displaystyle{ {\vec{r}_{f}} = {-1.398m} + {0.1632m/s}{0.1s} = {-1.2348m} }[/math]
Step 12: State Answer
After 0.2 seconds, the mass is at a position of 1.23 m below the ceiling.
A Note on Iterations: While calculations can be performed manually, as above, it is advisable for more advanced problems that VPython or a similar program be used for such repetitive calculations in order to save time and reduce the likelihood of mathematical errors.
Middling Example
A semi-challenging problem, such as the one below, will often require you to perform vector calculations. However, the same steps as the easy example above still apply. Pay careful attention to signs as you solve these types of problems.
The simplest example of a spring mass system is one that moves in only one-direction.
Consider a massless spring of relaxed length 0.5 m with spring constant 1200 N/m attached to the ceiling. If a 1.4 kg mass is released with an initial velocity of <1.2, 0.8, -0.2> m/s at an initial position of <-0.3, 0.7, 0.2> m, what is the velocity of the block after 0.005 seconds? Consider the point of attachment on the spring to the ceiling to be the origin. Only one iteration is necessary.
Step 1: Calculate Initial Values
Begin by calculating the object's initial momentum and the sum of the forces acting on it.
The initial momentum is simply the product of the mass and velocity of the object.
[math]\displaystyle{ {\vec{p}_{i}} = {{m}\cdot\vec{v}_{i}} }[/math]
[math]\displaystyle{ {\vec{p}_{i}} = {{1.4kg}\cdot{\lt 1.2, 0.8, -0.2\gt m/s}} = {\lt 1.68, 1.12, -0.28\gt Ns} }[/math]
The forces acting on the mass are the gravitational force exerted by the Earth and the tension exerted by the spring.
[math]\displaystyle{ {\vec{F}_{grav}} = {m}{\lt 0, -g, 0\gt } }[/math]
[math]\displaystyle{ {\vec{F}_{grav}} = {1.4kg}\cdot{\lt 0, -9.8, 0\gt m/s/s} = {\lt 0, -13.72, 0\gt N} }[/math]
[math]\displaystyle{ {\vec{F}_{spring} = {-k}{(\vec{L}_{0}-\vec{L})}
\cdot \frac{\vec{L}}}{\vec{{{math|1={{!}}x{{!}}}}}} }[/math]
[math]\displaystyle{ {\vec{F}_{spring} = {-1200N/m}\cdot{\lt 0, 0, 0\gt m} - {\lt 0, -9.8, 0\gt m} = {20 N}} }[/math]
l = ball.pos - ceiling.pos s = l.mag - L0 lhat = l/l.mag fspring = ks * s * -lhat
The net force can be calculated by summing these two forces.
[math]\displaystyle{ {\vec{F}_{net}} = {\vec{F}_{grav} +{\vec{F}_{spring}}} }[/math]
[math]\displaystyle{ {\vec{F}_{net}} = {-9.8N} +{20 N} = {10.2} }[/math]
Step 3: Update Momentum (Iteration 1)
Using the momentum update formula, calculate the momentum of the mass at the end of the given time step.
[math]\displaystyle{ {\vec{p}_{f} = \vec{p}_{i} + \vec{F}_{net}{Δt}} }[/math]
[math]\displaystyle{ {\vec{p}_{f} = {0Ns} + {10.2N}\cdot{0.1s} = {1.02Ns}} }[/math]
Step 4: Update Velocity (Iteration 1)
[math]\displaystyle{ {\vec{v}_{f} = \vec{v}_{i} + \frac{\vec{F}_{net}}{m}}{Δt} }[/math]
[math]\displaystyle{ {\vec{v}_{f} = {0m/s} + \frac{1.02N}{1 kg}}{0.1s} = {0.102m/s} }[/math]
Step 5: Update Position (Iteration 1)
Using the position update formula, calculate the position of the mass at the end of the given time step. The average velocity used below is the velocity of the mass at the end of the time step. As with all simplifying assumptions, this measurement is not exact. However, it allows for a close enough approximation that the results derived are still valid.
[math]\displaystyle{ {\vec{r}_{f}} = {\vec{r}_{i} + \vec{v}_{avg}{Δt}} }[/math]
[math]\displaystyle{ {\vec{r}_{f}} = {-1.5m} + {0.102m/s}{0.1s} = {-1.398m} }[/math]
Step 6: Update Time (Iteration 1)
[math]\displaystyle{ {\vec{t}_{f}} = {\vec{t}_{i}} + {Δt} }[/math]
[math]\displaystyle{ {\vec{t}_{f}} = {0s} + {0.1s} = {0.1s} }[/math]
Step 7: Repeat Calculations
Repeat the above calculations using the "Iteration Round 1 Final Values" (calculated above) as the "Iteration Round 2 Initial Values".
Step 8: Update Forces (Iteration 2)
The gravitational force on the mass remains constant, and does not need to be recalculated.
[math]\displaystyle{ {\vec{F}_{spring}} = {-kx} }[/math]
[math]\displaystyle{ {\vec{F}_{spring}} = {-40N/m}\cdot{1.0m-1.398m} = {15.92 N} }[/math]
[math]\displaystyle{ {\vec{F}_{net}} = {\vec{F}_{grav} +{\vec{F}_{spring}}} }[/math]
[math]\displaystyle{ {\vec{F}_{net}} = {-9.8N} +{15.92 N} = {6.12N} }[/math]
Step 9: Update Momentum (Iteration 2)
[math]\displaystyle{ {\vec{p}_{f} = \vec{p}_{i} + \vec{F}_{net}{Δt}} }[/math]
[math]\displaystyle{ {\vec{p}_{f} = {1.02Ns} + {6.12N}\cdot{0.1s} = {1.632Ns}} }[/math]
Step 10: Update Velocity (Iteration 2)
[math]\displaystyle{ {\vec{v}_{f} = \vec{v}_{i} + \frac{\vec{F}_{net}}{m}}{Δt} }[/math]
[math]\displaystyle{ {\vec{v}_{f} = {0m/s} + \frac{1.632N}{1 kg}}{0.1s} = {0.1632m/s} }[/math]
Step 11: Update Position (Iteration 2)
[math]\displaystyle{ {\vec{r}_{f}} = {\vec{r}_{i} + \vec{v}_{avg}{Δt}} }[/math]
[math]\displaystyle{ {\vec{r}_{f}} = {-1.398m} + {0.1632m/s}{0.1s} = {-1.2348m} }[/math]
Step 12: State Answer
After 0.2 seconds, the mass is at a position of 1.23 m below the ceiling.
Difficult Example
Very difficult iterative motion problems likely require several calculation loops to be performed. Thus, it is recommended that a computer program, such as VPython, is used to solve these. For general instructions on how to do this, please see above in this article, under the heading A Computational Model.
Connectedness
- How is this topic connected to something that you are interested in?
- How is it connected to your major?
- Is there an interesting industrial application?
See also
Are there related topics or categories in this wiki resource for the curious reader to explore? How does this topic fit into that context?
Further reading
Books, Articles or other print media on this topic
External links
Interactive Spring-Mass Simulator
References
This section contains the the references you used while writing this page